Library
System
Creating a library system using Object Oriented Programming (OOP) principles written in C++
Usage
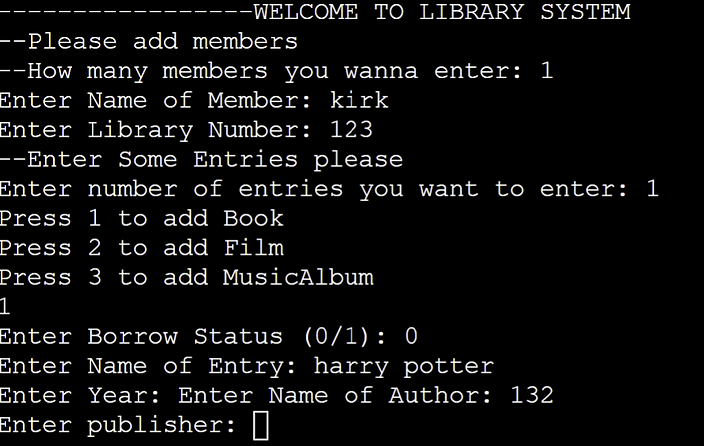
01.
Case where librarian wishes to add members to the library system, and is able add more than 1 member with required details of ID number and Name
03.
Now that the items have been entered into the library, the librarian can now issue an item to a library member
05.
In the case that information of stored items is incorrect, such as the entry name, the item will also fail to be issued
02.
The librarian is prompted to add entries to the Library System, and is able to add more than 1 entry, and can add a Book, Film, or Music Album. Can also store information on whether the items have been borrowed or not as requested
04.
In the case that the member is not part of the library, the librarian will not be able to issue an item.
:)
Catalogue class contains all the entries.
A clear functionality has been provided through adding entries, adding members, removing members, and issuing items.
All headers have been called from in the cpp file.
I have also overridden the virtual functions (polymorphism) in order to print all the details for the administrator.
For every class, default and parameterized constructors, as well as setters and getters were used to apply the best standard OOP coding practice, as well as sufficient commenting for additional developers to be able to adjust the code accordingly.
Full code found below
Main:
#include"Functionalities.h"
int main() {
cout << "-----------------WELCOME TO LIBRARY SYSTEM\n";
cout << "--Please add members for the Library\n";
cout << "--How many members would you like to enter? : ";
int num_members;
cin >> num_members;
LibrarySystem librar;
for (int i = 0; i < num_members; i++) {
librar.addMember();
}
cout << "--Enter some entries please\n";
librar.aDdEntry();
librar.issueItem();
return 0;
}
Functionalities.cpp:
#include "Functionalities.h"
//Default constructor
member::member() {
}
//Parameterised Constructor
member::member(string name, string number) {
//Assigning the parameter values to the attributes
this->name = name;
this->libraryNumber = number;
}
//Getter function of get name
string member::getMemberName() {
return this->name; //Returning the name
}
//Getter of the Library number of the member
string member::getLibraryNumber() {
return this->libraryNumber;
}
//Setter
void member::setlibraryNumber(string n) {
libraryNumber = n; //Setting value
}
//Setter
void member::setMemberName(string n) {
name = n; //Setting value
}
//Print the details of the member
void member::printMember() {
cout << "Name of Member: " << name << endl; //print name
cout << "Library Number of the Member: " << libraryNumber << endl; //print LibraryNumber
}
//Take input from user to set the values
//It is a helper function
void member::inputMember() {
cout << "Enter Name of Member: ";
cin >> name; //input name
cout << "Enter Library Number: ";
cin >> libraryNumber; //input Library Number
}
///////////////////////////////////////////// Entry Class ///////////////////////////////////////////////////
//Default Constructor
Entry::Entry() {
borrowed = false;
}
//Parameterised Constructor
Entry::Entry(bool borrowed, string borrowedBy, string name, string year) {
//Assigning all the values
this->borrowed = borrowed;
this->borrowedBy = borrowedBy;
this->name = name;
this->year = year;
}
//Getter
bool Entry::getBorrowed() {
return this->borrowed;//Returning the borrowed
}
//Getter
string Entry::getBorrowedby() {
return this->borrowedBy; //returning the borrowed by
}
//Getter
string Entry::getName() {
return this->name; //returning the name
}
//Getter
string Entry::getYear() {
return this->year;
}
//Setter
void Entry::setBorrowed(bool b) {
this->borrowed = b; //setting parameter value to attribute
}
//Setter
void Entry::setBorrowedby(string b) {
this->borrowedBy = b;//setting parameter value to attribute
}
//Setter
void Entry::setName(string name) {
this->name = name;//setting parameter value to attribute
}
//Setter
void Entry::setYear(string year) {
this->year = year;//setting parameter value to attribute
}
//virtual function (Polymorphism)
//print function to print all the details
void Entry::printDetails() {
//printing details
cout << "Borrowed: " << borrowed << endl;
cout << "Borrowed By: " << borrowedBy << endl;
cout << "Name: " << name << endl;
cout << "Year: " << year << endl;
}
//virtual function (Polymorphism)
//function to set the values of attributes
void Entry::inputDetails() {
cout << "Enter Borrow Status (0/1) [0 if not borrowed, 1 for borrowed]: ";
cin >> borrowed;
if (borrowed) {
cout << "Enter Name of the Member who borrowed: ";
cin >> borrowedBy;
}
cout << "Enter Name of Entry: ";
cin >> name;
cout << "Enter Year: ";
cin >> year;
}
////////////////////////////////////////////// MusicAlbum Class //////////////////////////////////////////////
//default constructor
MusicAlbum::MusicAlbum() :Entry() {
}
//parameterised constructor
MusicAlbum::MusicAlbum(bool borrowed, string borrowedBy, string name, string year, string artist, string recordLabel)
:Entry(borrowed, borrowedBy, name, year) {
this->artist = artist;
this->recordLabel = recordLabel;
}
//Getter
string MusicAlbum::getArtist() {
return this->artist; //returning value
}
//Getter
string MusicAlbum::getRecodlabel() {
return this->recordLabel; //returning value
}
//Setter
void MusicAlbum::setArtist(string a) {
this->artist = a; //setting up the parameter value
}
//Setter
void MusicAlbum::setRecodlabel(string r) {
this->recordLabel = r; //setting up the parameter value
}
//override the virtual function (polymorphism)
//printing the details
void MusicAlbum::printDetails() {
Entry::printDetails();
cout << "Artist: " << artist << endl;
cout << "Record Label: " << recordLabel << endl;
}
//override the virtual function (polymorphism)
//input from the user
void MusicAlbum::inputDetails() {
Entry::inputDetails();
cout << "Enter Name of Artist: ";
cin >> artist;
cout << "Enter Record Label: ";
cin >> recordLabel;
}
/////////////////////////////// book class ////////////////////////////////////////
Book::Book() :Entry() {//default constructor
}
//parameterised constructor
Book::Book(bool borrowed, string borrowedBy, string name, string year, string author, string publisher, string edition)
//setting up all the values
:Entry(borrowed, borrowedBy, name, year) {
this->author = author;
this->edition = edition;
this->publisher = publisher;
}
//Getter
string Book::getAuthor() {
return this->author;//returning value
}
//Getter
string Book::getEdition() {
return this->edition;//returning value
}
//Getter
string Book::getPublisher() {
return this->publisher;//returning value
}
//Setter
void Book::setAuthor(string a) {
this->author = a;//setting up the parameter value
}
//Setter
void Book::setPublisher(string p) {
this->publisher = p;//setting up the parameter value
}
//Setter
void Book::setEdition(string e) {
this->edition = e;//setting up the parameter value
}
//override the virtual function (polymorphism)
//printing the details
void Book::printDetails(){
Entry::printDetails();
cout << "Author: " << author << endl;
cout << "Edition: " << edition << endl;
cout << "Publisher: " << publisher << endl;
}
//override the virtual function (polymorphism)
//input from the user
void Book::inputDetails() {
Entry::inputDetails();
cout << "Enter Name of Author: ";
cin >> author;
cout << "Enter Publisher: ";
cin >> publisher;
cout << "Enter Edition: ";
cin >> edition;
}
/////////////////////////////////////// Film Class //////////////////////////////////////////
//Default constructor
Film::Film() :Entry() {
}
//parameterised constructor
Film::Film(bool borrowed, string borrowedBy, string name, string year, string director, string language)
:Entry(borrowed, borrowedBy, name, year) {
this->director = director;
this->language = language;
}
//Setter
void Film::setDirector(string d) {
director = d;
}
//Setter
void Film::setLanguage(string l) {
language = l;
}
//Getter
string Film::getDirector() {
return this->director;
}
//Getter
string Film::getLanguage() {
return this->language;
}
//override the virtual function (polymorphism)
//printing the details
void Film::printDetails() {
Entry::printDetails();
cout << "Director: " << director << endl;
cout << "Language: " << language << endl;
}
//override the virtual function (polymorphism)
//input from the user
void Film::inputDetails(){
Entry::inputDetails();
cout << "Enter Name of Director: ";
cin >> director;
cout << "Enter Language of Film: ";
cin >> language;
}
/////////////////////////////////// Catalogue Class //////////////////////////////////////////
Catalogue::Catalogue() { //Default constructor
}
//Parameterised Constructor
Catalogue::Catalogue(vector<Film> film, vector<Book> book, vector<MusicAlbum> musicAlbum) {
this->book = book;
this->film = film;
this->musicAlbum = musicAlbum;
}
//Function to add the entry
void Catalogue::addEntry() {
/// <summary>
/// printing the menu first to that the user can properly tell the system what they want to do
/// Then according to the option selected by the user, all the relevant information is gathered by the user and then the program
/// proceeds accordingly
/// </summary>
cout << "Press 1 to add Book\n";
cout << "Press 2 to add Film\n";
cout << "Press 3 to add MusicAlbum\n";
int option;
cin >> option;
if (option == 1) {
Book b;
b.inputDetails();
book.push_back(b);
}
if (option == 2) {
Film f;
f.inputDetails();
film.push_back(f);
}
if (option == 3) {
MusicAlbum m;
m.inputDetails();
musicAlbum.push_back(m);
}
}
//Function is there to provide ease to Admin if they want to remove the entry from the library
void Catalogue::removeEntry() {
//printing the menu first so that the user can tell the system what to remove
cout << "Press 1 to remove Book\n";
cout << "Press 2 to remove Film\n";
cout << "Press 3 to remove MusicAlbum\n";
int option;
cin >> option;
if (option == 1) {
string n;
bool found = false;
cout << "Enter Name of the book: "; //taking the name of the entry
cin >> n;
for (int i = 0; i < book.size(); i++) {
//checking the name if it is an already stored entry
if (book[i].getName() == n) { //enters this in case the required entry is found
found = true;
//removing the required entry
book[i] = book[book.size() - 1];
book.pop_back();
cout << "Removed Successfully\n";
}
}
if (!found) { //case handling if the entry does not exist
cout << "No such Book Exists in record\n";
}
}
if (option == 2) {
string n;
bool found = false;
cout << "Enter Name of the Film: ";//taking the name of the entry
cin >> n;
for (int i = 0; i < film.size(); i++) {
//checking the name in the already stored entry
if (film[i].getName() == n) {//enters this in case the required entry is found
found = true;
film[i] = film[film.size() - 1];
film.pop_back();
cout << "Removed Successfully\n";
}
}
if (!found) {//case handling if the entry does not exist
cout << "No such Film Exists in record\n";
}
}
if (option == 3) {
string n;
bool found = false;
cout << "Enter Name of the Music Album: ";//taking the name of the entry
cin >> n;
for (int i = 0; i < musicAlbum.size(); i++) {
//checking the name if it is an already stored entry
if (musicAlbum[i].getName() == n) {//enters this in case the required entry is found
found = true;
musicAlbum[i] = musicAlbum[musicAlbum.size() - 1];
musicAlbum.pop_back();
cout << "Removed Successfully\n";
}
}
if (!found) {//case handling if entry does not exist
cout << "No such Music Album Exists in record\n";
}
}
}
//function to issue the entries
bool Catalogue::setIssue(string n, string m) {
//menu display
cout << "Press 1 to issue Book\n";
cout << "Press 2 to issue Film\n";
cout << "Press 3 to issue MusicAlbum\n";
int option;
cin >> option;
if (option == 1) {
bool found = false;
for (int i = 0; i < book.size(); i++) {
if (book[i].getName() == n) {
found = true;
book[i] = book[book.size() - 1];
book.pop_back();
return true;
}
}
return found;
}
if (option == 2) {
bool found = false;
for (int i = 0; i < film.size(); i++) {
if (film[i].getName() == n) {
found = true;
film[i].setBorrowedby(m);
film[i].setBorrowed(1);
return true;
}
}
return found;
}
if (option == 3) {
bool found = false;
for (int i = 0; i < musicAlbum.size(); i++) {
if (musicAlbum[i].getName() == n) {
found = true;
musicAlbum[i].setBorrowedby(m);
musicAlbum[i].setBorrowed(1);
return true;
}
}
return found;
}
return 0;
}
////////////////////////////////////// Library class /////////////////////////////////////////
//Default constructor
LibrarySystem::LibrarySystem() {
}
// Parameterised constructor
LibrarySystem::LibrarySystem(vector<member> members,
Catalogue entries) {
this->members = members;
this->entries = entries;
}
void LibrarySystem::addMember() {
member m;
m.inputMember();
members.push_back(m);
}
void LibrarySystem::aDdEntry() {
cout << "Enter number of entries you want to enter: ";
int c = 0;
cin >> c;
for (int i = 0; i < c; i++) {
entries.addEntry();
}
}
void LibrarySystem::removeMember() {
cout << "Enter Library Number: ";
string n;
bool found = false;
}
void LibrarySystem::issueItem() {
cout << "-----Welcome to Issue the Entries Section\n";
cout << "Enter Name of Entry: ";
string n;
cin >> n;
cout << "Enter Library Number of the Member: ";
string m;
cin >> m;
bool a = false, b = false;
for (int i = 0; i < members.size(); i++) {
if (m == members[i].getLibraryNumber()) {
a = true;
if (entries.setIssue(n, m) == true) {
cout << "Issued Successfully\n";
b = true;
}
}
}
if (!a) {
cout << "This member is not a part of the library\n";
}
if (!b) {
cout << "Issued fail\n";
}
}
Functionalities.h:
#include <iostream>
#include <string>
#include <vector>
using namespace std;
//Member class to store the Information of member of library
class member {
string name; //to store name of member
string libraryNumber; //to store the library number of the member
public:
//Default constructor
member();
//Parameterised Constructor
member(string name, string number);
//Getter function of get name
string getMemberName();
//Getter of the Library number of the member
string getLibraryNumber();
//Setter
void setlibraryNumber(string n);
//Setter
void setMemberName(string n);
//print the details of member
void printMember();
//Take input from user to set the values
//It is a helper function
void inputMember();
};
//Entry class to store the information of entry
class Entry {
bool borrowed; // to store if a book is borrowed or not
string borrowedBy; //contains the library number of member who borrowed this entry
string name; //store name of entry
string year; //store the year
public:
//Default Constructor
Entry();
//Parameterised Constructor
Entry(bool borrowed, string borrowedBy, string name, string year);
//Getter
bool getBorrowed();
//Getter
string getBorrowedby();
//Getter
string getName();
//Getter
string getYear();
//Setter
void setBorrowed(bool b);
//Setter
void setBorrowedby(string b);
//Setter
void setName(string name);
//Setter
void setYear(string year);
//virtual function (Polymorphism)
//print function to print all the details
virtual void printDetails();
//virtual function (Polymorphism)
//function to set the values of attributes
virtual void inputDetails();
};
class MusicAlbum : public Entry { //this class is inherited from the Entry class
//stores the name of artist
string artist;
string recordLabel; //stores record label
public:
//default constructor
MusicAlbum();
//parameterised constructor
MusicAlbum(bool borrowed, string borrowedBy, string name, string year, string artist, string recordLabel);
//Getter
string getArtist();
//Getter
string getRecodlabel();
//Setter
void setArtist(string a);
//Setter
void setRecodlabel(string r);
//overrides the virtual function (polymorphism)
//printing the details
void printDetails()override;
//overrides the virtual function (polymorphism)
//input from the user
void inputDetails()override;
};
class Book :public Entry {//this class is inherited from the Entry class
//stores author
string author;
string publisher; //stores publisher
string edition; //stores the edition
public:
Book();
//parameterised constructor
Book(bool borrowed, string borrowedBy, string name, string year, string author, string publisher, string edition);
//Getter
string getAuthor();
//Getter
string getEdition();
//Getter
string getPublisher();
//Setter
void setAuthor(string a);
//Setter
void setPublisher(string p);
//Setter
void setEdition(string e);
//overrides the virtual function (polymorphism)
//printing the details
void printDetails()override;
//overrides the virtual function (polymorphism)
//input from the user
void inputDetails()override;
};
class Film : public Entry {
string director;
string language;
public:
//Default constructor
Film();
//parameterised constructor
Film(bool borrowed, string borrowedBy, string name, string year, string director, string language);
//Setter
void setDirector(string d);
//Setter
void setLanguage(string l);
//Getter
string getDirector();
//Getter
string getLanguage();
//overrides the virtual function (polymorphism)
//printing the details
void printDetails()override;
//overrides the virtual function (polymorphism)
//input from the user
void inputDetails()override;
};
//This is the class to store the information of all the entries of the library
class Catalogue {
vector<Film> film; //to store the films
vector<Book> book; //to store the books
vector<MusicAlbum> musicAlbum; //to store the music Albums
public:
//Default constructor
Catalogue();
//Parameterised Constructor
Catalogue(vector<Film> film, vector<Book> book, vector<MusicAlbum> musicAlbum);
//Function to add the entry
void addEntry();
//Function is there to provide ease to admin if they want to remove the entry from the library
void removeEntry();
//function to issue the entries
bool setIssue(string n, string m);
};
//Final class to manage the overall library system
class LibrarySystem {
vector<member> members; //to store members
Catalogue entries; //to store the entries
public:
//default constructor
LibrarySystem();
LibrarySystem(vector<member> members,
Catalogue entries);
void addMember();
void aDdEntry();
void removeMember();
void issueItem();
};